BLOG
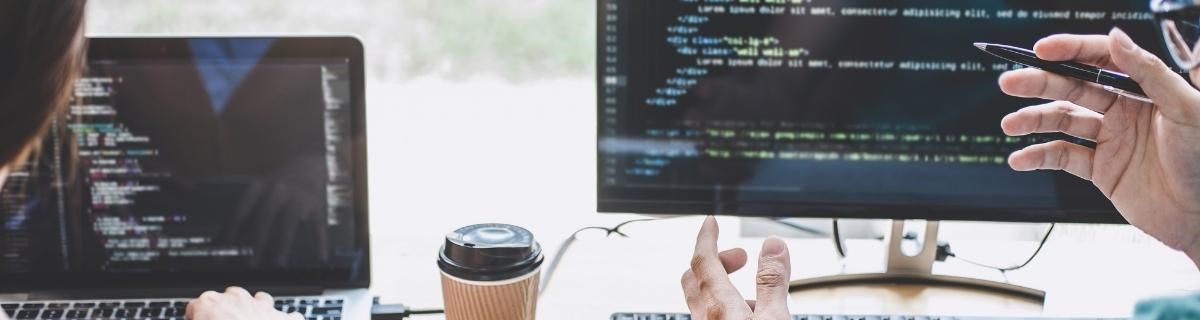
When and How to use the Futurism Gem with Ruby on Rails
The basic use case for Futurize is to “lazy-load” sections of a web page. You may want to do this for example if you have a complex application, and you want to load sections of the page after the main or important content has loaded. Or perhaps you have too much data, but it's all-important. You need to load it all, but you don't want page load times to be 30 seconds.
In the past, you might create a div with a spinner, then on document ready, make an ajax call to load that content. Futurize is the same basic idea only instead of an ajax call, you now pass that data over ActionCable.
What's even nicer is that you can do this without even thinking about it. So long as action cable is set up and ready, you are basically good to go.
Use Cases for the Futurize Gem
A great example for this might be a Hotel that is trying to book rooms for customers as they call in. Imagine a calendar interface with 90 days worth of booking data, and each day you need to show both open rooms at levels (suites, rooms, penthouses) and reservations.
You want each day to show all booked reservations.
You want each reservation to show last names and room numbers.
You want each day to show the number of rooms at a level that are booked/open/unavailable.
You want to show today + the next 90 days.
Already, as a developer, your brain kicks in: “That's a lot of data”, How can I even make that look good? Never mind looking good; how can I make it usable?”
What you come up with is a table with 10 columns and 9 rows. Each cell is 1 day, giving you 90 days. Each cell can be clicked on and will expand to show the full details required, but the mini-view will just show the date, booked, open, and remaining rooms at each room level. About 5 lines of text.
Now you start to structure your views and come up with something like:
<div id="date-grid">
<% (Date.today..(Date.today + 90.days)).in_groups_of(10).each do |days| %>
<div class="row">
<% days.each do |day| %>
<div class="column">
<div id="<%= day.to_i %>" class="day-cell">
<div class="always-show">
<%= render partial: "day", locals: { date: day } %>
</div>
<div class="hidden">
<%= render partial: 'details', locals: { date: day } %>
</div>
</div>
</div>
<% end %>
</div>
<% end %>
</div>
And you wire up some javascript so that when “.day-cell” is clicked its child “.hidden” is shown. Great. The interface is loved, and it should really help out, except, it takes forever to load. 45 seconds or more. There's just too much information, especially in the details section. The expanded idea is really loved, but 200 rooms means 200 names, 200 room numbers, and 200 ”is the room paid for” checks (don't forget about scope creep).
So, you segment out the code a bit and you get the 90 days of summary information showing pretty fast. But there's just nothing for it. 90 days * 200 rooms * 2-3 lookups, it's going to take a while.
This is where futurism can really come in. Again, in the past, ajax would have done this. But now instead of 90 HTTP requests were going to transfer over that same data, via WebSocket.
All we have to do as a developer is change
<%= render partial: 'details', locals: { date: day } %>
To
<%= futurisim partial: 'details', extends: 'div', locals: { date: day } %>
Now when the page loads, only the summary information is fetched, rendered, and transmitted. At some later point, the `details` partial will be rendered and sent via ActionCable. We don’t care when. Most importantly, users feel a faster load time because the page has the critical information in 2 seconds instead of 45 seconds.
YOU MAY ALSO LIKE
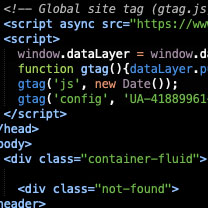
May 29, 2019 - By Dave S.
Rails Authorization: Comparing CanCan and Pundit
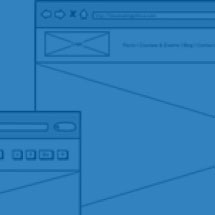
June 12, 2019 - By Dave S.
Rails Soft Delete: Comparing Paranoia and Discard
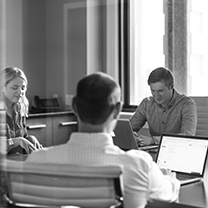